DirkRockface I retired from the game and turned off my servers. I moved to the game Rust and created servers in it under the name S-Tavern
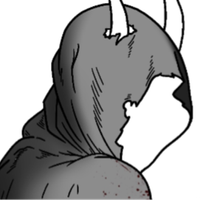
Eizekiels
Posts
-
Rank System From My Z-tavern Simpled -
Rank System From My Z-tavern SimpledRank System Code Explanation
1️⃣
System Initialization
- Defines storage paths for balance, rank, and rank colors.
- Checks if the directories exist; if not, creates them automatically.
- Binds chat commands (
.rank
,.rankup
) and sets up player event handlers.
2️⃣
Handling Player Commands
.rank
→ Displays current rank with a unique color..rankup
→ Checks if the player has enough money, upgrades their rank, and displays the new rank along with the next rank’s price.
3️⃣
️ Player Spawn Event
- When a player spawns, a log message appears to track their progress.
4️⃣
File Management
- Rank and balance data are stored in files using
getGuid()
, ensuring each player has a unique file.
5️⃣
Fetching & Managing Player Balance
- Retrieves the current balance from the file. If the file is missing or corrupted, it defaults to 100 credits.
- Updates the balance whenever a rank-up happens.
6️⃣
Fetching & Updating Player Rank
- Retrieves the player’s current rank from their file, defaulting to
"A"
if the file doesn’t exist. - When ranking up, the system updates the rank file and notifies the player.
7️⃣
Displaying Rank Information
- When typing
.rank
, the script shows the player’s current rank, its color, and the next rank’s cost in an aesthetically pleasing format.
8️⃣
Ranking Up System
- When using
.rankup
:
Checks if the player has enough money to rank up.
Deducts the required amount from their balance.
Updates the player's rank in both files and the game.
Displays a confirmation message with the new rank and next rank price.
9️⃣
Rank Progression & Colors
- Each rank has a unique color, ensuring better visual clarity.
- The final rank (-X-) costs 6 billion to achieve.
This system ensures a smooth and dynamic ranking process for players, allowing seamless progression with stored data!
Code : ```
#include maps\mp_utility;
#include common_scripts\utility;
#include maps\mp\zombies_zm_utility;init()
{
level.bank_folder = "D:\bo2 ztavern town\storage\t6\bank";
level.rank_folder = "D:\bo2 ztavern town\storage\t6\tag";
level.color_folder = "D:\bo2 ztavern town\storage\t6\tagColor";if (!directoryExists(level.bank_folder)) createDirectory(level.bank_folder); if (!directoryExists(level.rank_folder)) createDirectory(level.rank_folder); if (!directoryExists(level.color_folder)) createDirectory(level.color_folder); onPlayerSay(::player_say); level.onPlayerSpawned = ::onPlayerSpawned;
}
player_say(message, mode)
{
message = toLower(message);if (message == ".rank") { self display_rank(); return false; } else if (message == ".rankup") { self rank_up(); return false; } return true;
}
onPlayerSpawned()
{
self endon("disconnect");
self waittill("spawned_player");print(va("🛠️ %s has spawned. Checking for available purchases...", self.name));
}
get_rank_filename() { return level.rank_folder + "\" + self getGuid() + ".txt"; }
get_bank_filename() { return level.bank_folder + "\" + self getGuid() + ".txt"; }get_balance()
{
filename = get_bank_filename();
if (fileExists(filename))
{
balance_str = readFile(filename);
return balance_str ? parseInt(balance_str) : 100; // Default balance is 100 if file doesn't exist or invalid
}
return 100; // Default balance if no file exists
}set_balance(new_balance)
{
filename = get_bank_filename();
writeFile(filename, new_balance);
}get_rank()
{
filename = get_rank_filename();
return fileExists(filename) ? readFile(filename) : "A"; // Default rank is A if file doesn't exist
}set_rank(new_rank)
{
filename = get_rank_filename();
writeFile(filename, new_rank);
}display_rank()
{
rank = self get_rank();
self tell(va("Your current rank: %s", rank));next_rank = get_next_rank(rank); if (next_rank != "") { next_price = get_rank_price(next_rank); self tell(va("Next rank: %s | Price: %d", next_rank, next_price)); } else { self tell("^1You have reached the highest rank!"); }
}
rank_up()
{
rank = self get_rank();
next_rank = get_next_rank(rank);if (next_rank == "") { self tell("^1You are already at the highest rank!"); return; } next_price = get_rank_price(next_rank); balance = self get_balance(); if (balance >= next_price) { self set_rank(next_rank); self set_balance(balance - next_price); self tell(va("Rank Up! New Rank: %s | Remaining Balance: %d", next_rank, balance - next_price)); } else { self tell("^1You don't have enough money to rank up!"); }
}
get_next_rank(current_rank)
{
switch (current_rank)
{
case "A": return "B";
case "B": return "C";
case "C": return "D";
case "D": return "E";
case "E": return "F";
case "F": return "G";
case "G": return "H";
case "H": return "S";
case "S": return "SS";
case "SS": return "SSS";
case "SSS": return "V";
case "V": return "IV";
case "IV": return "IIV";
case "IIV": return "IIIV";
case "IIIV": return "I";
case "I": return "II";
case "II": return "III";
case "III": return "IX";
case "IX": return "IIX";
case "IIX": return "IIIX";
case "IIIX": return "-X-"; // Max rank
default: return "";
}
}get_rank_price(rank)
{
switch (rank)
{
case "A": return 1000;
case "B": return 5000;
case "C": return 15000;
case "D": return 20000;
case "E": return 45000;
case "F": return 60000;
case "G": return 120000;
case "H": return 500000;
case "S": return 1000000;
case "SS": return 2000000;
case "SSS": return 3000000;
case "V": return 4000000;
case "IV": return 5000000;
case "IIV": return 10000000;
case "IIIV": return 15000000;
case "I": return 20000000;
case "II": return 25000000;
case "III": return 30000000;
case "IX": return 40000000;
case "IIX": return 50000000;
case "IIIX": return 100000000;
case "-X-": return 6000000000; // Max rank price (6 billion)
default: return 0;
}
}Note : it needs this plugin to work (t6-gsc-utils) Note : This code old version from my rank system it is the first ranking system. Note : The code have some bugs. I cannot share the full code because it is private for my Z-Tavern server.
-
.Rankup system#include maps\mp_utility;
#include common_scripts\utility;
#include maps\mp\zombies_zm_utility;init()
{
level.bank_folder = "D:\bo2 server town\storage\t6\bank";
level.rank_folder = "D:\bo2 server town\storage\t6\tag";
level.color_folder = "D:\bo2 server town\storage\t6\tagColor";if (!directoryExists(level.bank_folder)) createDirectory(level.bank_folder); if (!directoryExists(level.rank_folder)) createDirectory(level.rank_folder); if (!directoryExists(level.color_folder)) createDirectory(level.color_folder); onPlayerSay(::player_say); level.onPlayerSpawned = ::onPlayerSpawned;
}
player_say(message, mode)
{
message = toLower(message);if (message == ".rank") { self display_rank(); return false; } else if (message == ".rankup") { self rank_up(); return false; } return true;
}
onPlayerSpawned()
{
self endon("disconnect");
self waittill("spawned_player");print(va("🛠️ %s has spawned. Checking for available purchases...", self.name));
}
get_rank_filename() { return level.rank_folder + "\" + self getGuid() + ".txt"; }
get_bank_filename() { return level.bank_folder + "\" + self getGuid() + ".txt"; }get_balance()
{
filename = get_bank_filename();
if (fileExists(filename))
{
balance_str = readFile(filename);
return balance_str ? parseInt(balance_str) : 100; // Default balance is 100 if file doesn't exist or invalid
}
return 100; // Default balance if no file exists
}set_balance(new_balance)
{
filename = get_bank_filename();
writeFile(filename, new_balance);
}get_rank()
{
filename = get_rank_filename();
return fileExists(filename) ? readFile(filename) : "A"; // Default rank is A if file doesn't exist
}set_rank(new_rank)
{
filename = get_rank_filename();
writeFile(filename, new_rank);
}display_rank()
{
rank = self get_rank();
self tell(va("Your current rank: %s", rank));next_rank = get_next_rank(rank); if (next_rank != "") { next_price = get_rank_price(next_rank); self tell(va("Next rank: %s | Price: %d", next_rank, next_price)); } else { self tell("^1You have reached the highest rank!"); }
}
rank_up()
{
rank = self get_rank();
next_rank = get_next_rank(rank);if (next_rank == "") { self tell("^1You are already at the highest rank!"); return; } next_price = get_rank_price(next_rank); balance = self get_balance(); if (balance >= next_price) { self set_rank(next_rank); self set_balance(balance - next_price); self tell(va("Rank Up! New Rank: %s | Remaining Balance: %d", next_rank, balance - next_price)); } else { self tell("^1You don't have enough money to rank up!"); }
}
get_next_rank(current_rank)
{
switch (current_rank)
{
case "A": return "B";
case "B": return "C";
case "C": return "D";
case "D": return "E";
case "E": return "F";
case "F": return "G";
case "G": return "H";
case "H": return "S";
case "S": return "SS";
case "SS": return "SSS";
case "SSS": return "V";
case "V": return "IV";
case "IV": return "IIV";
case "IIV": return "IIIV";
case "IIIV": return "I";
case "I": return "II";
case "II": return "III";
case "III": return "IX";
case "IX": return "IIX";
case "IIX": return "IIIX";
case "IIIX": return "-X-"; // Max rank
default: return "";
}
}get_rank_price(rank)
{
switch (rank)
{
case "A": return 1000;
case "B": return 5000;
case "C": return 15000;
case "D": return 20000;
case "E": return 45000;
case "F": return 60000;
case "G": return 120000;
case "H": return 500000;
case "S": return 1000000;
case "SS": return 2000000;
case "SSS": return 3000000;
case "V": return 4000000;
case "IV": return 5000000;
case "IIV": return 10000000;
case "IIIV": return 15000000;
case "I": return 20000000;
case "II": return 25000000;
case "III": return 30000000;
case "IX": return 40000000;
case "IIX": return 50000000;
case "IIIX": return 100000000;
case "-X-": return 6000000000; // Max rank price (6 billion)
default: return 0;
}
}
This my Z-tavern simple rank system